Let’s solve LeetCode 1929 – Concatenation of Array problem. It’s from the Arrays section on LeetCode, and as usual, we’ll tackle it using C# today.
Before we jump into the code, let’s understand the problem statement.
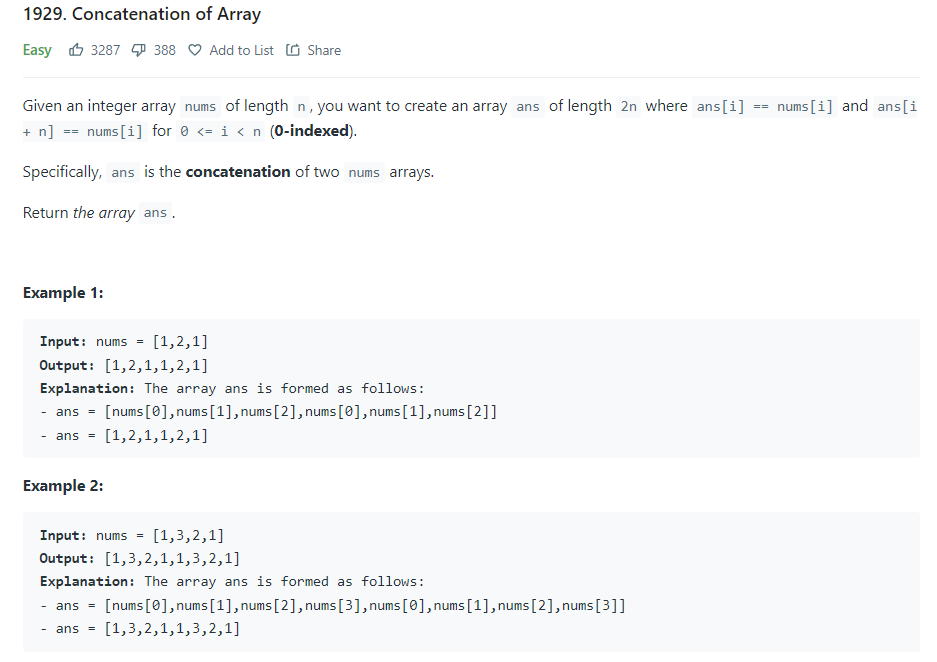
This problem challenges you to create a new array by “duplicating” a given integer array. The resulting array should have twice the length of the original, with each element from the original array appearing twice consecutively (That’s the key to solve this challenge).
Understanding the Problem:
- We are given an integer array
nums
of lengthn
. - We need to create a new array
ans
of length2n
(twice the length of the original). - The elements in the new array
ans
should be arranged such thatans[i] == nums[i]
andans[i + n] == nums[i]
for all indicesi
from 0 ton-1
.
In simpler terms, the new array ans
is the concatenation of two copies of the original array nums
.
Alright, let’s start designing our solution:
1) We need to create a new array ans
that can hold twice the number of elements as the original array nums
.
public class Solution {
public int[] GetConcatenation(int[] nums) {
int[] ans = new int[nums.Length*2];
}
}
2) We need to iterate through each element in the original array nums
and copy it to the new array ans
at two specific positions ( i and i + nums.Length).
public class Solution {
public int[] GetConcatenation(int[] nums) {
int[] ans = new int[nums.Length*2];
for(int i = 0; i < nums.Length; i++){
ans[i] = nums[i];
ans[i + nums.Length] = nums[i];
}
}
}
- The line
ans[i] = nums[i]
copies the element at indexi
fromnums
to the corresponding indexi
in theans
array. This ensures the first half of theans
array is a copy of the originalnums
. - The line
ans[i + nums.Length] = nums[i]
is the key part for concatenation. It copies the same element at indexi
fromnums
to an index that isnums.Length
positions ahead of the current indexi
in theans
array. This effectively places a copy of each element fromnums
in the second half of theans
array.
3) Now we just need to return ans
after the loop!
public class Solution {
public int[] GetConcatenation(int[] nums) {
int[] ans = new int[nums.Length*2];
for(int i = 0; i < nums.Length; i++){
ans[i] = nums[i];
ans[i + nums.Length] = nums[i];
}
return ans;
}
}
This solution iterates through the original array, copies each element to its corresponding position in the first half of the new array, and then copies it again to the calculated position in the second half, resulting in the desired concatenation.
This is the result for this solution:
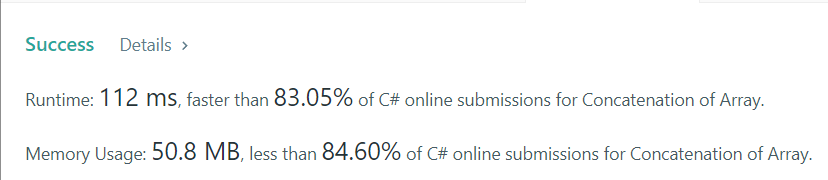